AES-hamming
复盘一道cybrics的密码题。
题目
题目提到数据发生了错误,但需要我们进行纠错,纠错用上了汉明码。
题目源码如下
1 | #!/usr/bin/python3.7 |
(附图如上)
赛中回想
题目大致意思理清了和使用的算法都明晰了,但出现了几个疑问:
- 到底错误出现在原图上还是传输中;
- 这道题到底要不要爆破;
- 15字节内最多一个错误到底怎么用。
然后,就没啦。
赛后复盘
直接粘复盘记录过来。
题目提示主要是用hamming码纠错。
目前情况如下:
汉明码纠错将一组120bit即15字节转换为16字节,能纠正一位错误或检测两位错误2.对出错位置进行了分析,发现共有0xc8a组,其中错误有128处,有一组错误并自动纠错71组,能检测出两组错误的有57组,且所有错误均分布于0x404之前。
目前能识读的文段为G3nTl3_4Nd_5oFt_3RroR5_R4T3}
想到,既然题目说每15个字节最多1处错误,能不能直接按字节枚举错误的划分方式,这样的话,在同一段汉明码内如果出现了两个错误,必然分别出现在两段之内,似乎可以降低复杂度。啊啊啊写不出来wwww
复盘1:看到WM说通过枚举坏块然后去掉的方式可做,但这种方法根本就没用上题目信息啊啊啊,整合一下出来是这个图
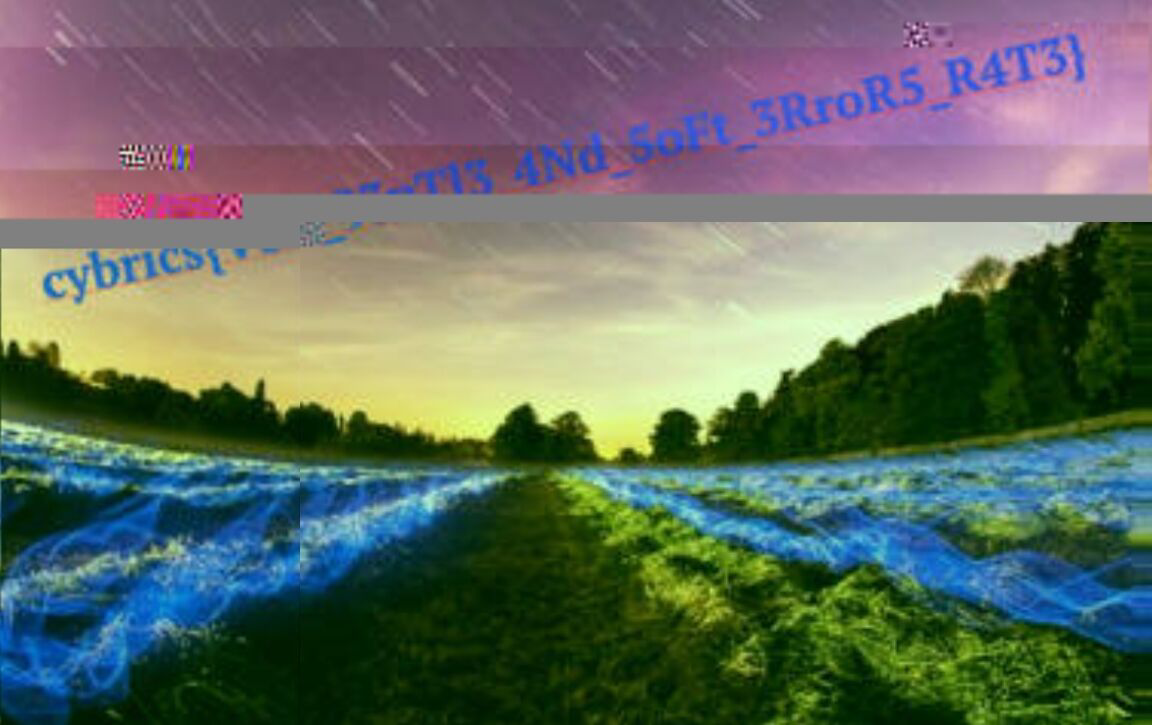
不太认WM的这个做法。
复盘2,原来题目考点是CBC比特反转。
首先,错误到底发生在了哪。答:发生在了密文传输的过程中,本质还是在考AES
其次,错误是怎么产生的。答:原文提到降低到每15字节最多发生一个比特翻转,这句话的实际表达居然是每十六字节一个错误。
再次,怎么判定错误发生在传输而不是明文本身。答:检查出现双错误的字节,发现有连续三个的双错误字节,而其最多只能含有3*16//15+2=5个错误,说明明文本身错误这个假设不成立。
再者,为什么才来复盘。答:意识到是AES本身的错误后,这几天一直在重学CBC翻转攻击,然后开始纠正,但因为对封装好的AES认识不到位,把前边的密文直接作为iv使用导致了奇怪的错误,用了两天重写脚本用统一的iv解决的。
脚本:
1 |
|
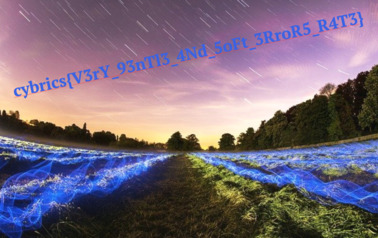
(所以WM那帮人是怎么看出来这个V3rY的)